Indicates whether a loading bar should be shown or hidden below the search bar
boolean
false
markdown
The CommonMark string to be rendered.
string
-
metadata
The Detail.Metadata to be rendered in the right side area
React.ReactNode
-
navigationTitle
The main title for that view displayed in Raycast
string
Command title
You can specify custom image dimensions by adding a raycast-width and raycast-height query string to the markdown image. For example: 
You can also specify a tint color to apply to an markdown image by adding a raycast-tint-color query string. For example: 
You can now render LaTeX in the markdown. We support the following delimiters:
Inline math: \(...\) and \begin{math}...\end{math}
Display math: \[...\], $$...$$ and \begin{equation}...\end{equation}
Detail.Metadata
A Metadata view that will be shown in the right-hand-side of the Detail.
Use it to display additional structured data about the main content shown in the Detail view.
Example
import { Detail } from"@raycast/api";// Define markdown here to prevent unwanted indentation.constmarkdown=`# Pikachu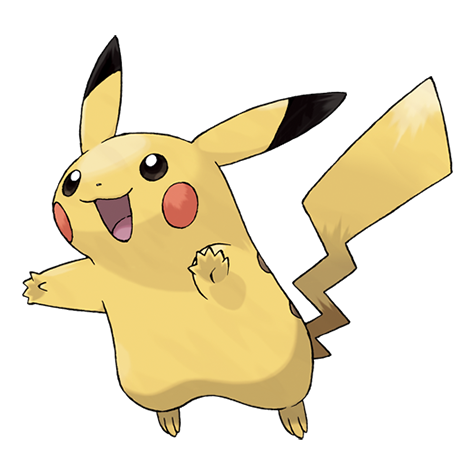Pikachu that can generate powerful electricity have cheek sacs that are extra soft and super stretchy.`;exportdefaultfunctionMain() {return (<Detail markdown={markdown} navigationTitle="Pikachu" metadata={ <Detail.Metadata> <Detail.Metadata.Label title="Height" text={`1' 04"`} /> <Detail.Metadata.Label title="Weight" text="13.2 lbs"/> <Detail.Metadata.TagList title="Type"> <Detail.Metadata.TagList.Item text="Electric" color={"#eed535"} /> </Detail.Metadata.TagList> <Detail.Metadata.Separator /> <Detail.Metadata.Link title="Evolution" target="https://www.pokemon.com/us/pokedex/pikachu" text="Raichu"/> </Detail.Metadata> }/> );}
Props
Prop
Description
Type
Default
children*
The elements of the Metadata view.
React.ReactNode
-
Detail.Metadata.Label
A single value with an optional icon.
Example
import { Detail } from"@raycast/api";// Define markdown here to prevent unwanted indentation.constmarkdown=`# Pikachu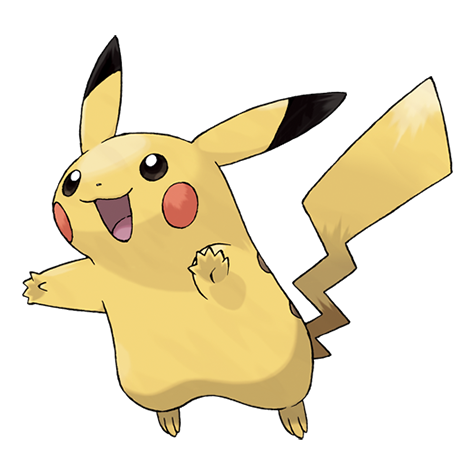Pikachu that can generate powerful electricity have cheek sacs that are extra soft and super stretchy.`;exportdefaultfunctionMain() {return (<Detail markdown={markdown} navigationTitle="Pikachu" metadata={ <Detail.Metadata> <Detail.Metadata.Label title="Height" text={`1' 04"`} icon="weight.svg"/> </Detail.Metadata> }/> );}
import { Detail } from"@raycast/api";// Define markdown here to prevent unwanted indentation.constmarkdown=`# Pikachu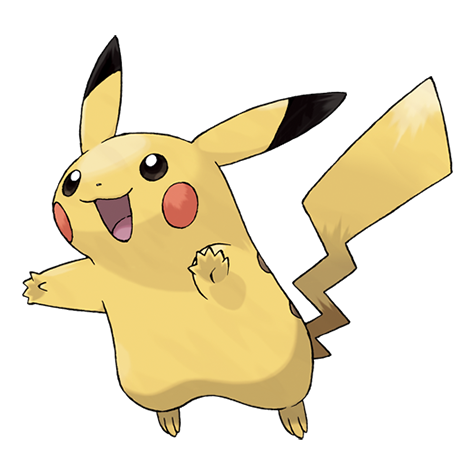Pikachu that can generate powerful electricity have cheek sacs that are extra soft and super stretchy.`;exportdefaultfunctionMain() {return (<Detail markdown={markdown} navigationTitle="Pikachu" metadata={ <Detail.Metadata> <Detail.Metadata.Link title="Evolution" target="https://www.pokemon.com/us/pokedex/pikachu" text="Raichu"/> </Detail.Metadata> }/> );}
import { Detail } from"@raycast/api";// Define markdown here to prevent unwanted indentation.constmarkdown=`# Pikachu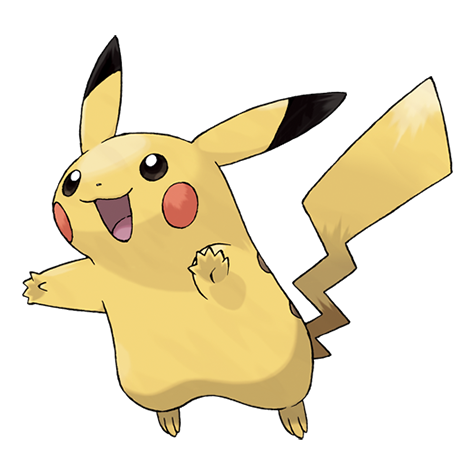Pikachu that can generate powerful electricity have cheek sacs that are extra soft and super stretchy.`;exportdefaultfunctionMain() {return (<Detail markdown={markdown} navigationTitle="Pikachu" metadata={ <Detail.Metadata> <Detail.Metadata.TagList title="Type"> <Detail.Metadata.TagList.Item text="Electric" color={"#eed535"} /> </Detail.Metadata.TagList> </Detail.Metadata> }/> );}
Props
Prop
Description
Type
Default
children*
The tags contained in the TagList.
React.ReactNode
-
title*
The title shown above the item.
string
-
Detail.Metadata.TagList.Item
A Tag in a Detail.Metadata.TagList.
Props
Prop
Description
Type
Default
color
Changes the text color to the provided color and sets a transparent background with the same color.
The optional tag text. Required if the tag has no icon.
string
-
onAction
Callback that is triggered when the item is clicked.
() => void
-
Detail.Metadata.Separator
A metadata item that shows a separator line. Use it for grouping and visually separating metadata items.
import { Detail } from"@raycast/api";// Define markdown here to prevent unwanted indentation.constmarkdown=`# Pikachu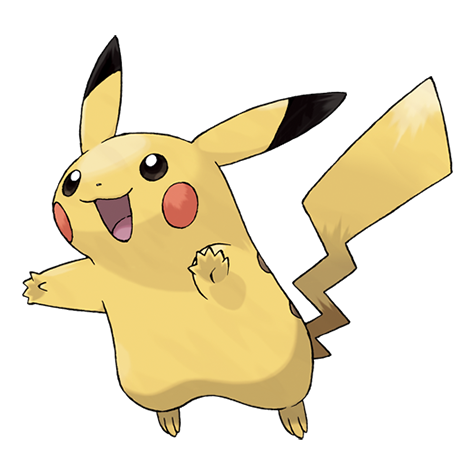Pikachu that can generate powerful electricity have cheek sacs that are extra soft and super stretchy.`;exportdefaultfunctionMain() {return (<Detail markdown={markdown} navigationTitle="Pikachu" metadata={ <Detail.Metadata> <Detail.Metadata.Label title="Height" text={`1' 04"`} /> <Detail.Metadata.Separator /> <Detail.Metadata.Label title="Weight" text="13.2 lbs"/> </Detail.Metadata> }/> );}